本博客日IP超过2000,PV 3000 左右,急需赞助商。
极客时间所有课程通过我的二维码购买后返现24元微信红包,请加博主新的微信号:xttblog2,之前的微信号好友位已满,备注:返现
受密码保护的文章请关注“业余草”公众号,回复关键字“0”获得密码
所有面试题(java、前端、数据库、springboot等)一网打尽,请关注文末小程序
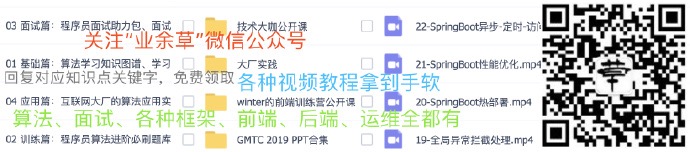
腾讯云】1核2G5M轻量应用服务器50元首年,高性价比,助您轻松上云
前面有一篇已经写过 Shiro的 Realm了。传送门:Shiro 的 Realm。本文我们在继续深入学习自定义Realm功能。
现实中的权限关系
即用户-角色之间是多对多关系,角色-权限之间是多对多关系;且用户和权限之间通过角色建立关系;在系统中验证时通过权限验证,角色只是权限集合,即所谓的显示角色;其实权限应该对应到资源(如菜单、URL、页面按钮、Java方法等)中,即应该将权限字符串存储到资源实体中,但是目前为了简单化,直接提取一个权限表。该表包含一下内容:
用户实体包括:编号(id)、用户名(username)、密码(password)、盐(salt)、是否锁定(locked);是否锁定用于封禁用户使用,其实最好使用Enum字段存储,可以实现更复杂的用户状态实现。
角色实体包括:、编号(id)、角色标识符(role)、描述(description)、是否可用(available);其中角色标识符用于在程序中进行隐式角色判断的,描述用于以后再前台界面显示的、是否可用表示角色当前是否激活。
权限实体包括:编号(id)、权限标识符(permission)、描述(description)、是否可用(available);含义和角色实体类似不再阐述。
另外还有两个关系实体:用户-角色实体(用户编号、角色编号,且组合为复合主键);角色-权限实体(角色编号、权限编号,且组合为复合主键)。
drop table if exists sys_users; drop table if exists sys_roles; drop table if exists sys_permissions; drop table if exists sys_users_roles; drop table if exists sys_roles_permissions; create table sys_users ( id bigint auto_increment, username varchar(100), password varchar(100), salt varchar(100), locked bool default false, constraint pk_sys_users primary key(id) ) charset=utf8 ENGINE=InnoDB; create unique index idx_sys_users_username on sys_users(username); create table sys_roles ( id bigint auto_increment, role varchar(100), description varchar(100), available bool default false, constraint pk_sys_roles primary key(id) ) charset=utf8 ENGINE=InnoDB; create unique index idx_sys_roles_role on sys_roles(role); create table sys_permissions ( id bigint auto_increment, permission varchar(100), description varchar(100), available bool default false, constraint pk_sys_permissions primary key(id) ) charset=utf8 ENGINE=InnoDB; create unique index idx_sys_permissions_permission on sys_permissions(permission); create table sys_users_roles ( user_id bigint, role_id bigint, constraint pk_sys_users_roles primary key(user_id, role_id) ) charset=utf8 ENGINE=InnoDB; create table sys_roles_permissions ( role_id bigint, permission_id bigint, constraint pk_sys_roles_permissions primary key(role_id, permission_id) ) charset=utf8 ENGINE=InnoDB;
这里需要配合数据库进行操作,因此我们在pom文件中添加数据库支持。详细案例源代码下载地址见本文末尾。
主要逻辑在service层,实现基本的创建/删除权限。
public interface PermissionService { public Permission createPermission(Permission permission); public void deletePermission(Long permissionId); } public interface RoleService { public Role createRole(Role role); public void deleteRole(Long roleId); //添加角色-权限之间关系 public void correlationPermissions(Long roleId, Long... permissionIds); //移除角色-权限之间关系 public void uncorrelationPermissions(Long roleId, Long... permissionIds);// } public interface UserService { public User createUser(User user); //创建账户 public void changePassword(Long userId, String newPassword);//修改密码 public void correlationRoles(Long userId, Long... roleIds); //添加用户-角色关系 public void uncorrelationRoles(Long userId, Long... roleIds);// 移除用户-角色关系 public User findByUsername(String username);// 根据用户名查找用户 public Set<String> findRoles(String username);// 根据用户名查找其角色 public Set<String> findPermissions(String username); //根据用户名查找其权限 }
此处使用findByUsername、findRoles及findPermissions来查找用户名对应的帐号、角色及权限信息。之后的Realm就使用这些方法来查找相关信息。
UserServiceImpl关键代码如下:
public User createUser(User user) { //加密密码 passwordHelper.encryptPassword(user); return userDao.createUser(user); } public void changePassword(Long userId, String newPassword) { User user =userDao.findOne(userId); user.setPassword(newPassword); passwordHelper.encryptPassword(user); userDao.updateUser(user); }
在创建账户及修改密码时直接把生成密码操作委托给PasswordHelper。
PasswordHelper
public class PasswordHelper { private RandomNumberGenerator randomNumberGenerator = new SecureRandomNumberGenerator(); private String algorithmName = "md5"; private final int hashIterations = 2; public void encryptPassword(User user) { user.setSalt(randomNumberGenerator.nextBytes().toHex()); String newPassword = new SimpleHash( algorithmName, user.getPassword(), ByteSource.Util.bytes(user.getCredentialsSalt()), hashIterations).toHex(); user.setPassword(newPassword); } }
之后的CredentialsMatcher需要和此处加密的算法一样。user.getCredentialsSalt()辅助方法返回username+salt。为了节省篇幅,对于DAO/Service的接口及实现,具体请参考源码。
自定义Realm
RetryLimitHashedCredentialsMatcher
package com.xttblog.shiro5.credentials; import net.sf.ehcache.CacheManager; import net.sf.ehcache.Ehcache; import net.sf.ehcache.Element; import org.apache.shiro.authc.AuthenticationInfo; import org.apache.shiro.authc.AuthenticationToken; import org.apache.shiro.authc.ExcessiveAttemptsException; import org.apache.shiro.authc.credential.HashedCredentialsMatcher; import java.util.concurrent.atomic.AtomicInteger; public class RetryLimitHashedCredentialsMatcher extends HashedCredentialsMatcher { private Ehcache passwordRetryCache; public RetryLimitHashedCredentialsMatcher() { CacheManager cacheManager = CacheManager.newInstance(CacheManager.class.getClassLoader().getResource("ehcache.xml")); passwordRetryCache = cacheManager.getCache("passwordRetryCache"); } @Override public boolean doCredentialsMatch(AuthenticationToken token, AuthenticationInfo info) { String username = (String)token.getPrincipal(); //retry count + 1 Element element = passwordRetryCache.get(username); if(element == null) { element = new Element(username , new AtomicInteger(0)); passwordRetryCache.put(element); } AtomicInteger retryCount = (AtomicInteger)element.getObjectValue(); if(retryCount.incrementAndGet() > 5) { //if retry count > 5 throw throw new ExcessiveAttemptsException(); } boolean matches = super.doCredentialsMatch(token, info); if(matches) { //clear retry count passwordRetryCache.remove(username); } return matches; } }
UserRealm
public class UserRealm extends AuthorizingRealm { private UserService userService = new UserServiceImpl(); protected AuthorizationInfo doGetAuthorizationInfo(PrincipalCollection principals) { String username = (String)principals.getPrimaryPrincipal(); SimpleAuthorizationInfo authorizationInfo = new SimpleAuthorizationInfo(); authorizationInfo.setRoles(userService.findRoles(username)); authorizationInfo.setStringPermissions(userService.findPermissions(username)); return authorizationInfo; } protected AuthenticationInfo doGetAuthenticationInfo(AuthenticationToken token) throws AuthenticationException { String username = (String)token.getPrincipal(); User user = userService.findByUsername(username); if(user == null) { throw new UnknownAccountException();//没找到帐号 } if(Boolean.TRUE.equals(user.getLocked())) { throw new LockedAccountException(); //帐号锁定 } //交给AuthenticatingRealm使用CredentialsMatcher进行密码匹配,如果觉得人家的不好可以在此判断或自定义实现 SimpleAuthenticationInfo authenticationInfo = new SimpleAuthenticationInfo( user.getUsername(), //用户名 user.getPassword(), //密码 ByteSource.Util.bytes(user.getCredentialsSalt()),//salt=username+salt getName() //realm name ); return authenticationInfo; } }
- UserRealm父类AuthorizingRealm将获取Subject相关信息分成两步:获取身份验证信息(doGetAuthenticationInfo)及授权信息(doGetAuthorizationInfo);
- doGetAuthenticationInfo获取身份验证相关信息:首先根据传入的用户名获取User信息;然后如果user为空,那么抛出没找到帐号异常UnknownAccountException;如果user找到但锁定了抛出锁定异常LockedAccountException;最后生成AuthenticationInfo信息,交给间接父类AuthenticatingRealm使用CredentialsMatcher进行判断密码是否匹配,如果不匹配将抛出密码错误异常IncorrectCredentialsException;另外如果密码重试此处太多将抛出超出重试次数异常ExcessiveAttemptsException;在组装SimpleAuthenticationInfo信息时,需要传入:身份信息(用户名)、凭据(密文密码)、盐(username+salt),CredentialsMatcher使用盐加密传入的明文密码和此处的密文密码进行匹配。
- doGetAuthorizationInfo获取授权信息:PrincipalCollection是一个身份集合,因为我们现在就一个Realm,所以直接调用getPrimaryPrincipal得到之前传入的用户名即可;然后根据用户名调用UserService接口获取角色及权限信息。
java 测试用例
测试用例代码见com.xttblog.shiro5.realm.UserRealmTest,包含了:登录成功、用户名错误、密码错误、密码超出重试次数、有/没有角色、有/没有权限的测试。
本文源代码下载链接:http://pan.baidu.com/s/1eSCCt7k 密码:czvl
最后,欢迎关注我的个人微信公众号:业余草(yyucao)!可加作者微信号:xttblog2。备注:“1”,添加博主微信拉你进微信群。备注错误不会同意好友申请。再次感谢您的关注!后续有精彩内容会第一时间发给您!原创文章投稿请发送至532009913@qq.com邮箱。商务合作也可添加作者微信进行联系!
本文原文出处:业余草: » Shiro 自定义Realm